Programming Examples
Write a program to interface a temperature sensor (LM35) and water Nozzle with the Arduino.
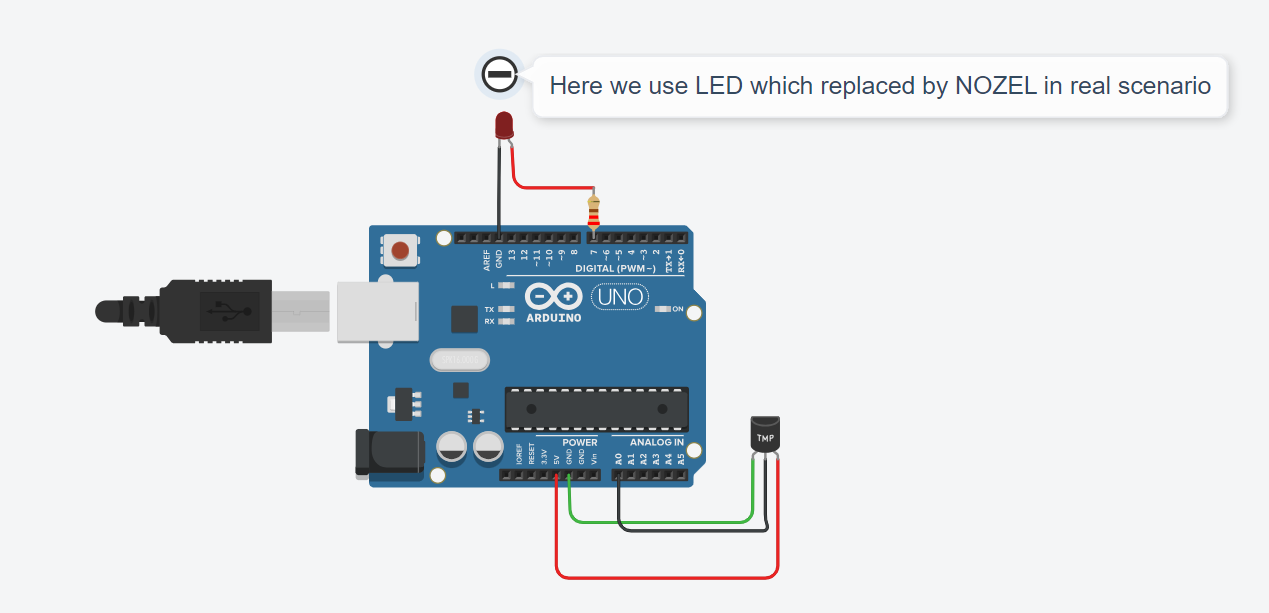
Write a program to interface a temperature sensor (LM35) and water Nozzle with the Arduino.
A) make the water flow from Nozzle, when the room temperature is above 34 degree Celsius when temperature falls below 18 degree Celsius.
B) Also put a condition of maximum on time of the Nozzle as 2 minutes.
// Pin connected to the LM35 temperature sensor
const int temperaturePin = A0;
// Pin connected to the relay or transistor controlling the water nozzle
const int nozzlePin = 7;
// Temperature thresholds for water flow control
const int upperThreshold = 34; // Celsius
const int lowerThreshold = 18; // Celsius
// Maximum on time for the water nozzle in milliseconds (2 minutes)
const unsigned long maxOnTime = 2 * 60 * 1000; // 2 minutes in milliseconds
unsigned long nozzleStartTime = 0; // Variable to store the start time of water flow
void setup() {
pinMode(temperaturePin, INPUT);
pinMode(nozzlePin, OUTPUT);
digitalWrite(nozzlePin, LOW); // Ensure the nozzle is initially turned off
}
void loop() {
// Read the temperature from the LM35 sensor
int temperature = readTemperature();
// Check temperature conditions for water flow
if (temperature > upperThreshold && nozzleStartTime == 0) {
// Room temperature is above 34 degrees, and nozzle is not already ON
startWaterFlow();
} else if (temperature < lowerThreshold && nozzleStartTime != 0) {
// Room temperature is below 18 degrees, and nozzle is currently ON
stopWaterFlow();
}
// Check maximum on time condition
if (nozzleStartTime != 0 && millis() - nozzleStartTime >= maxOnTime) {
stopWaterFlow(); // Turn off the nozzle if the maximum on time is reached
}
}
int readTemperature() {
// Read analog value from LM35 temperature sensor
int sensorValue = analogRead(temperaturePin);
// Convert analog value to temperature in Celsius
float temperatureCelsius = (sensorValue * 0.48876) - 50.0;
return int(temperatureCelsius);
}
void startWaterFlow() {
digitalWrite(nozzlePin, HIGH); // Turn on the water nozzle
nozzleStartTime = millis(); // Record the start time of water flow
}
void stopWaterFlow() {
digitalWrite(nozzlePin, LOW); // Turn off the water nozzle
nozzleStartTime = 0; // Reset the start time
}
Output