Programming Examples
Design a night lamp using a LDR and a LED using Arduino Uno and find out the time delays associated in keeping ON the lamp
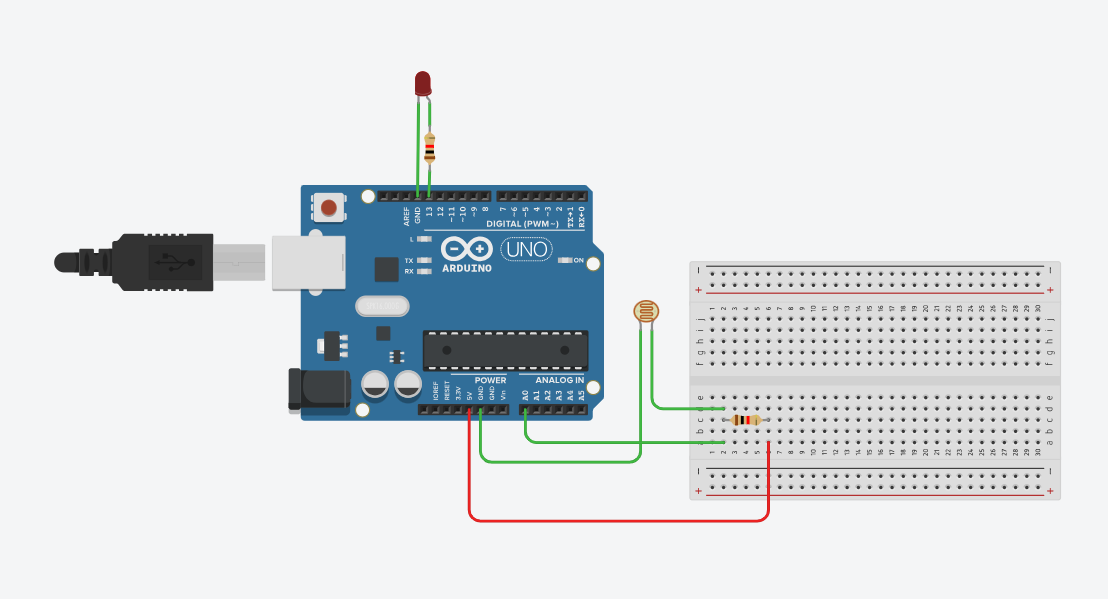
Design a night lamp using a LDR and a LED using Arduino Uno and find out the time delays associated in keeping ON the lamp
Components Needed:
- Arduino Uno
- LDR (Light Dependent Resistor)
- LED
- Resistor (for LED current limiting)
- Breadboard and jumper wires
Circuit Connections:
Connect the components as follows:
- Connect one leg of the LDR to 5V on the Arduino.
- Connect the other leg of the LDR to analog pin A0 on the Arduino.
- Connect one leg of the resistor to the junction of the LDR and connect the other leg of the resistor to the ground (GND) on the Arduino.
- Connect the cathode (shorter leg) of the LED to digital pin 13 on the Arduino.
- Connect the anode (longer leg) of the LED to a current-limiting resistor, and the other end of the resistor to the ground (GND) on the Arduino.
const int LDR_PIN = A0; // LDR connected to analog pin A0
const int LED_PIN = 13; // LED connected to digital pin 13
int threshold = 500; // Adjust this threshold value based on ambient light conditions
unsigned long lampOnTime = 0;
unsigned long lampOffTime = 0;
void setup() {
pinMode(LED_PIN, OUTPUT);
Serial.begin(9600);
}
void loop()
{
int lightValue = analogRead(LDR_PIN);
if (lightValue < threshold && lampOnTime==0)
{
// Turn ON the LED when it's dark and record the start time
digitalWrite(LED_PIN, HIGH);
lampOnTime = millis(); // Record the start time
Serial.print("Lamp ON Time: ");
Serial.println(lampOnTime);
}
else if (lightValue >= threshold && lampOnTime>0)
{
// Turn OFF the LED when it's bright and record the stop time
digitalWrite(LED_PIN, LOW);
lampOffTime = millis(); // Record the stop time
Serial.print("Lamp OFF Time: ");
Serial.println(lampOffTime);
Serial.print("Lamp ON Delay Time: ");
Serial.println((lampOffTime - lampOnTime)/1000); // Convert milliseconds to seconds
Serial.print(" seconds");
lampOnTime = 0;
lampOffTime = 0;
}
}
Output