Programming Examples
Arduino program to interface security password with Arduino board to show green light for correct password and red light for incorrect password.
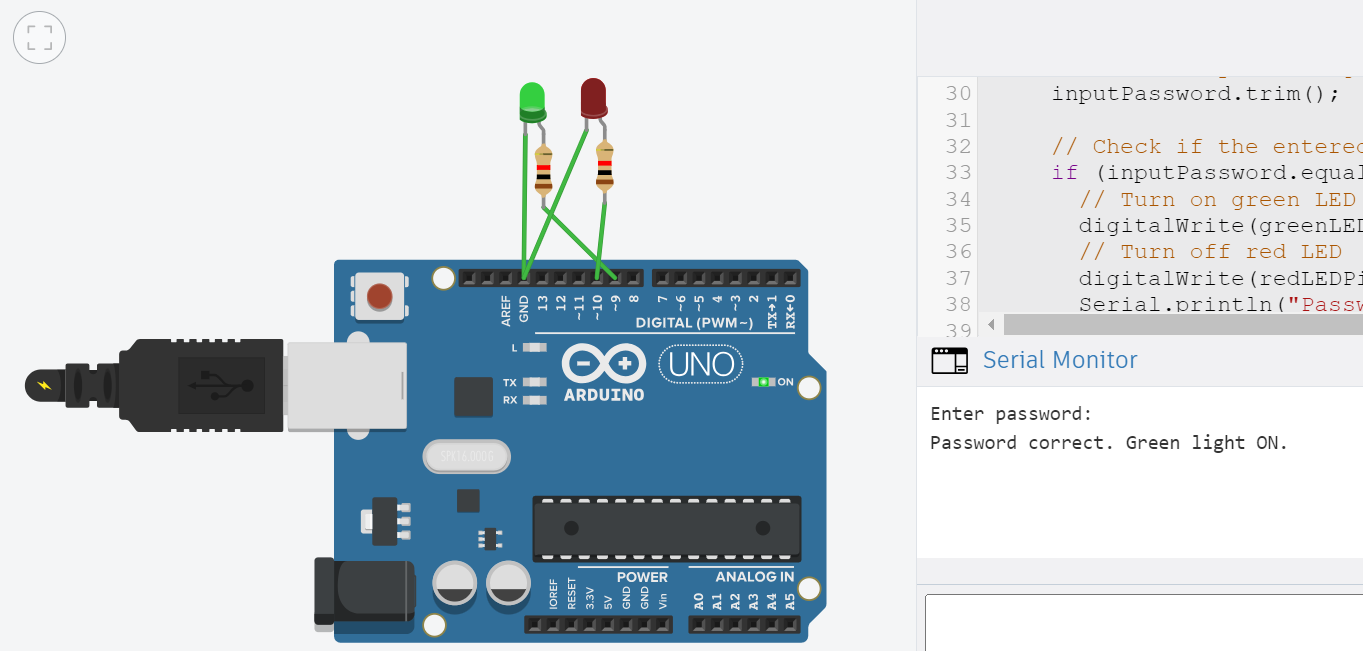
Write a program to interface security password with Arduino board to show green light for correct password and red light for incorrect password.
Solution
// Define pins for LEDs
const int greenLEDPin = 9;
const int redLEDPin = 10;
// Predefined correct password
String correctPassword = "1234";
void setup() {
// Initialize the LED pins as outputs
pinMode(greenLEDPin, OUTPUT);
pinMode(redLEDPin, OUTPUT);
// Initialize Serial communication at 9600 baud rate
Serial.begin(9600);
// Turn off both LEDs at start
digitalWrite(greenLEDPin, LOW);
digitalWrite(redLEDPin, LOW);
Serial.println("Enter password:");
}
void loop() {
// Check if data is available to read
if (Serial.available() > 0) {
// Read the incoming string
String inputPassword = Serial.readStringUntil('\n');
// Remove any trailing newline character
inputPassword.trim();
// Check if the entered password matches the correct password
if (inputPassword.equals(correctPassword)) {
// Turn on green LED
digitalWrite(greenLEDPin, HIGH);
// Turn off red LED
digitalWrite(redLEDPin, LOW);
Serial.println("Password correct. Green light ON.");
} else {
// Turn off green LED
digitalWrite(greenLEDPin, LOW);
// Turn on red LED
digitalWrite(redLEDPin, HIGH);
Serial.println("Password incorrect. Red light ON.");
}
}
}
Output