Programming Examples
Arduino application where pressing a button increases a counter value, which is then displayed on an LCD
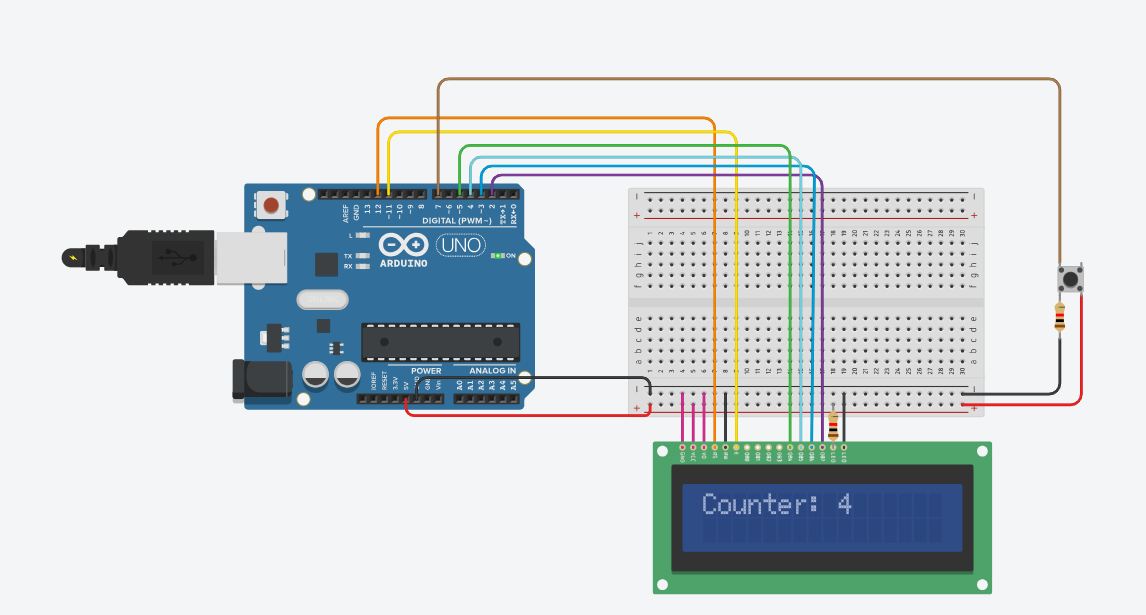
Arduino application where pressing a button increases a counter value, which is then displayed on an LCD, you would typically be working with hardware such as an Arduino. Below is an example of how you can achieve this using an Arduino with an LCD and a button.
Components Needed:
- Arduino board (e.g., Arduino Uno)
- LCD display (e.g., 16x2 LCD)
- Push button
- Resistors (e.g., 10k ohm for the button pull-down resistor)
- Breadboard and jumper wires
LCD Connections:
- VSS to GND
- VDD to 5V
- V0 to the middle pin of a 10k potentiometer (other pins to GND and 5V)
- RS to Arduino pin 12
- RW to GND
- E to Arduino pin 11
- D4 to Arduino pin 5
- D5 to Arduino pin 4
- D6 to Arduino pin 3
- D7 to Arduino pin 2
- A (LED Anode) to a 220 ohm resistor, then to 5V
- K (LED Cathode) to GND
Button Connections:
- One side of the button to Arduino pin 7
- Other side of the button to GND
- Pull-up resistor (10k ohm) from the button pin to 5V
Solution
#include <LiquidCrystal.h>
// Initialize the library with the numbers of the interface pins
LiquidCrystal lcd(12, 11, 5, 4, 3, 2);
// Pin for the button
const int buttonPin = 7;
// Counter variable
int counter = 0;
// Variable to store the button state
int buttonState = 0;
int lastButtonState = 0;
void setup() {
// Set up the LCD's number of columns and rows:
lcd.begin(16, 2);
// Print the initial counter value
lcd.print("Counter: 0");
// Set the button pin as input
pinMode(buttonPin, INPUT);
// Use internal pull-up resistor
digitalWrite(buttonPin, HIGH);
}
void loop() {
// Read the button state
buttonState = digitalRead(buttonPin);
// Check if the button is pressed
if (buttonState != lastButtonState) {
if (buttonState == LOW) {
// Increase the counter
counter++;
// Clear the previous value
lcd.clear();
// Print the updated counter value
lcd.print("Counter: ");
lcd.print(counter);
}
delay(50); // debounce delay
}
// Save the current button state for comparison in the next loop
lastButtonState = buttonState;
}
Output
Explanation:
- Library Import: LiquidCrystal.h is included for LCD operations.
- LCD Initialization: LiquidCrystal lcd(12, 11, 5, 4, 3, 2); initializes the LCD with the specified pins.
- Button Setup: The button is connected to pin 7 and set as an input. The internal pull-up resistor is enabled by setting the pin to HIGH.
- Counter Initialization: The counter variable is initialized to 0.
- LCD Setup: lcd.begin(16, 2); sets up the LCD with 16 columns and 2 rows.
- Initial Display: The initial counter value (0) is displayed on the LCD.
- Main Loop: In the loop function, the button state is read and compared to the last state to detect button presses. If the button is pressed, the counter is incremented, and the new value is displayed on the LCD.
- Debouncing: A small delay (delay(50);) is added to debounce the button press.